Shortcuts Manager for Android
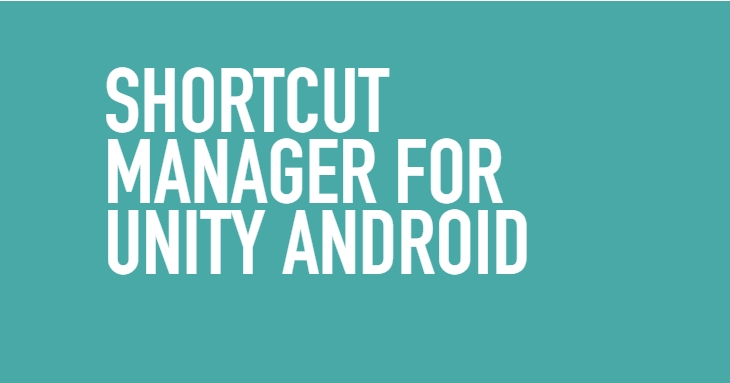
This package let you define dynamic shortcuts to perform specific actions in your app. These shortcuts can be displayed in a supported launcher and help your users quickly start common or recommended tasks within your app.
Basic functionality:
- Add dynamic Shortcut
- Update already existing one (short label / long label/ icon / action & rank)
- Remove existing Shortcuts
- Request Pin Shortcut
- Enable / Disable Shortcuts.
You can learn more about Shortcuts in Android here: Shortcuts
Download
You can download demo apk file using this link: Demo APK
Installation process
- Download the package from Package Manager
- Import it in your project
- Download Google’s Unity Jar Resolver from: Unity Jar Resolver and import in your project. After that resolve all dependencies by using: Assets -> External Dependency Manager -> Android Resolver -> Resolve or Force Resolve
- Add ShortcutsManager prefab from Prefabs folder to your scene.
- And that’s it, you can start coding!
Here you will learn how to Add, Update, Remove and Pin dynamic shortcuts to your app.
Add Shortcut
public const string OPEN_SCREEN = "screen_to_be_opened";
public void AddProfileShortcut () {
// Instance of UnityPlayerActivity
var activity = ShortcutsManager.Instance.GetCurrentAndroidActivity ();
// The actual Shortcut object
var profileShortcut = new Shortcut (
"user_profile_id", // shortcut id
"Profile", // shortcut short label
"Open profile and update your statistics!", // shortcut long label
"account_box", // icon name
0 // rank / order
);
// Intent object which will be 'executed' when
// the user click on the shortcut
var profileIntent = new Intent ()
// Set intent action.
.SetAction (IntentActions.ACTION_VIEW)
// Set intent class name.
.SetClassName (activity, ShortcutsManager.UNITY_PLAYER_ACTIVITY)
// Set intent flags
.SetFlags (IntentFlags.FLAG_ACTIVITY_SINGLE_TOP)
// Put int extra to the intent in order to be able
// to detect which screen we should open when the user
// clicks the shortcut.
.PutExtra (OPEN_SCREEN, (int) Screens.PROFILE);
// Tell the ShortcutManager to add the shortcut with
// the intent which should be executed when the shortcut
// is clicked.
ShortcutsManager.Instance.AddDynamicShortcut (
profileShortcut,
profileIntent
);
}
Update Shortcut
// Id of the shortcut which you want to update.
var id = "profile_id";
// If shortcut with this id does not exist, GetShortcutById will return null.
// Otherwise it will return valid shortcut object.
var shortcut = ShortcutsManager.Instance.GetShortcutById (id);
if (shortcut != null) {
// Update short label
shortcut.SetShortLabel ("New Short Label");
// Update long label
shortcut.SetLongLabel ("New Long Label");
// Update icon name
shortcut.SetIconName ("New Icon Name");
// Update rank
shortcut.SetRank (1);
// Pass the shortcut object to the the manager for update.
ShortcutsManager.Instance.UpdateShortcut (shortcut);
}
You can update an array of shortcuts together using:
var shortcutsArray = new Shortcut[size];
// Populate the array, get all shortcurs which you want to update
// in batch. Update all of them and pass to ShortcutsManager.
ShortcutsManager.Instance.UpdateShortcuts (shortcutsArray);
Or update the Shortcut with it’s Intent together:
var shortcut = ShortcutsManager.Instance.GetShortcutById (id);
if (shortcut != null) {
// ... Update shortcut data
var intent = new Intent();
// ... Update intent
// Pass shortcut and intent object to ShortcutsManager to update.
ShortcutsManager.Instance.UpdateShortcut (shortcut, intent)
}
Remove Shortcut
var shortcutId = "id";
// To remove a shortcut just call RemoveShortcutById.
ShortcutsManager.Instance.RemoveShortcutById(shortcutId);
You can remove shortcuts in batch by id too:
var shortcutIds = new string[size];
// Populate the array with ids of all shortcuts which
// you want to remove and pass the array to ShortcutsManager.
ShortcutsManager.Instance.RemoveShortcutsById();
If you want to remove all dynamic shortcuts you can just call:
ShortcutsManager.Instance.RemoveAllDynamicShortcuts();
Pin Shortcut
If you want to pin a shortcut you can do it easily by using:
var shortcutId = "id";
ShortcutsManager.Instance.RequestPinShortcutById(shortcutId);
Or by passing a Shortcut object and an Intent:
var id = "shortcut_id";
var shortcut = ShortcutsManager.Instance.GetShortcutById (id);
var intent = new Intent();
// Set data, classname and etc to the intent object.
// Keep in mind that this will present the user a dialog
// to pin the shortcut and it will be pinned only if the
// user approves that.
ShortcutsManager.Instance.RequestPinShortcut(shortcut, intent);
Testing
To test your app’s shortcuts, install your app on a device with a launcher that supports shortcuts. Then, perform the following actions:
- Long-tap on your app’s launcher icon to view the shortcuts that you’ve defined for your app.
- Tap and drag a shortcut to pin it to the device’s launcher.
Limitations
Although you can publish up to five shortcuts (static and dynamic shortcuts combined) at a time for your app, most launchers can only display four.
However, there is no limit to the number of pinned shortcuts to your app that users can create. Even though your app cannot remove pinned shortcuts, it can still disable them.
Although other apps can’t access the metadata within your shortcuts, the launcher itself can access this data.Therefore, these metadata should conceal sensitive user information.