Instagram Share Kit for Android
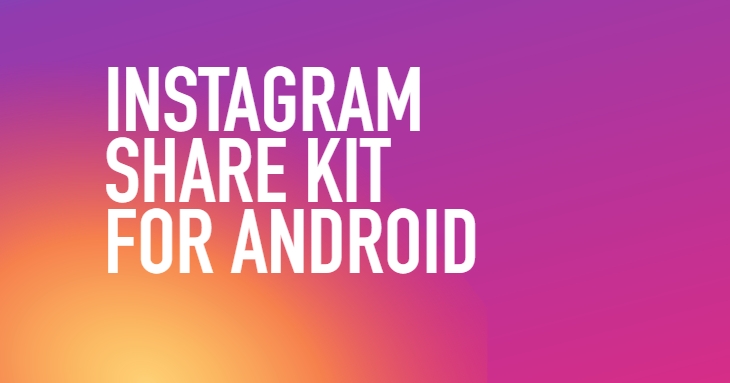
This Unity package lets you share your funny videos or pictures of your game directly to Instagram Android application.
- Download the package from Package Manager
- Import it in your project
- Download Google’s Unity Jar Resolver from: Unity Jar Resolver and import in your project. After that resolve all dependencies by using: Assets -> External Dependency Manager -> Android Resolver -> Resolve or Force Resolve
- And that’s it, you can start coding!
Sharing content using Instagram Share helper is easier than anything else, but before that there are a few things that needs to be done.
-
Open your main AndroidManifest.xml file in Assets/Plugins/Android/ folder and add this code inside tag:
<provider android:name="androidx.core.content.FileProvider" android:authorities="com.package.name.fileprovider" android:exported="false" android:grantUriPermissions="true"> <meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths"/> </provider>
Where com.package.name is the package name of your application.
-
Add this permission if it’s not already included in your AndroidManifest.xml file:
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
-
Last but not least add
<queries>
tag inside your manifest file:<queries> <package android:name="com.instagram.android" /> </queries>
Here is a sample AndroidManifest.xml file:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.unity3d.player" android:installLocation="preferExternal" android:theme="@android:style/Theme.NoTitleBar" android:versionCode="1" android:versionName="1.0">
<supports-screens android:smallScreens="true" android:normalScreens="true" android:largeScreens="true" android:xlargeScreens="true" android:anyDensity="true" />
<queries>
<package android:name="com.instagram.android" />
</queries>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<application android:icon="@drawable/app_icon" android:label="@string/app_name" android:debuggable="false">
<activity android:name="com.unity3d.player.UnityPlayerActivity" android:label="@string/app_name" android:configChanges="orientation|screenSize">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data android:name="unityplayer.UnityActivity" android:value="true" />
<meta-data android:name="unityplayer.ForwardNativeEventsToDalvik" android:value="true" />
</activity>
<provider android:name="androidx.core.content.FileProvider" android:authorities="com.hardartcore.instagramshare.fileprovider" android:exported="false" android:grantUriPermissions="true">
<meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths"/>
</provider>
</application>
</manifest>
And after the setup is complete you can use the helper class as:
public class DemoSceneScript : MonoBehaviour
{
// Share Image to Instagram
public void ShareImageToInstagram()
{
InstagramShareHelper.ShareImageToInstagram(filePath);
}
public void ShareVideoToInstagram()
{
InstagramShareHelper.ShareVideoToInstagram(filePath);
}
public void ShareImageAsInstagramStory()
{
InstagramShareHelper.ShareImageAsInstagramStory(filePath, null);
}
public void ShareVideoAsInstagramStory()
{
InstagramShareHelper.ShareVideoAsInstagramStory(filePath, null);
}
public void ShareImageWithGradientAsInstagramStory()
{
InstagramShareHelper.ShareImageWithGradientAsInstagramStory(filePath, "#02937B", "#1B64A9", null);
}
public void ShareBackgroundImageAndStickerAsInstagramStory()
{
InstagramShareHelper.ShareBackgroundImageAndStickerAsInstagramStory(backgroundImagePath, stickerPath, null);
}
}
Build Errors
If you encounter an issue: unexpected element <queries>
found in <manifest>
There is an easy fix for that: go to File -> Build Settings -> Player Settings -> Publishing Settings and select Custom Base Gradle Template. After that open the generated file baseProjectTemplate.gradle under Assets/Plugins/Android folder and change com.android.tools.build:gradle:***
to
Unity 2019.4+ -> com.android.tools.build:gradle:3.4.3
Unity 2021.3+ -> com.android.tools.build:gradle:4.0.1