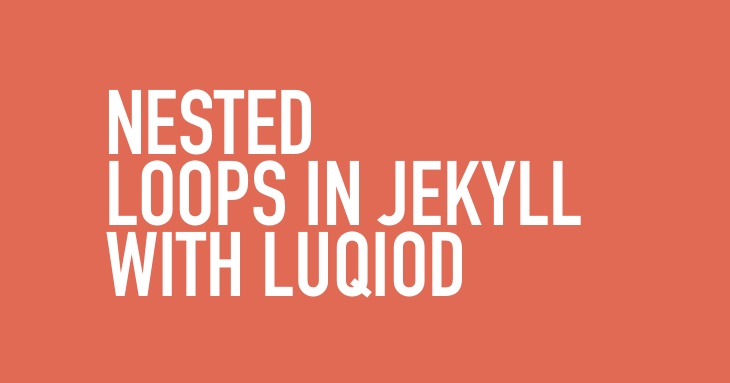
Unleashing the Power of Nested Loops in Jekyll with Liquid
Unleashing the Power of Nested Loops in Jekyll with Liquid Jekyll, a popular static site generator, is a fantastic tool for creating blogs, documentation, and websites. One of its key strengths lies in its use of Liquid, a templating language that allows for dynamic content generation. Today, we’ll explore a powerful technique within Liquid: nested for loops. This is particularly useful when dealing with hierarchical data, like a documentation structure with sections and sub-sections.
Let’s dive into a practical example. Imagine you’re building documentation for your game. You have a YAML file defining the structure of your documentation, including main sections and their sub-sections. This is where the power of nested loops shines.
---
layout: documentation
title: 'Game Documentation'
menu:
- section: 'Foundational Concepts'
url: 'foundational_concepts'
- section: 'Gameplay Mechanics'
url: 'gameplay_mechanics'
menu:
- section: 'Core Interactions'
url: 'core_interactions'
- section: 'Player Controls'
url: 'player_controls'
- section: 'Game Modes'
url: 'game_modes'
- section: 'AI Behavior'
url: 'ai_behaviour'
- section: 'Assets'
url: 'assets'
menu:
- section: 'Visual Assets'
url: 'visual_assets'
- section: 'Audio Assets'
url: 'audio_assets'
- section: '3D Models'
url: '3d_models'
- section: 'Technical Aspects'
url: 'technical_aspects'
menu:
- section: 'Performance Optimization'
url: 'performance_optimization'
- section: 'Memory Management'
url: 'memory_management'
- section: 'Development'
url: 'development'
menu:
- section: 'Code Style Guide'
url: 'code_style_guide'
- section: 'Testing Procedures'
url: 'testing_procedures'
---
This YAML defines the structure of our documentation. We have main sections like “Foundational Concepts”, “Development,” and “Technical Aspects.” Some of these sections, like “Assets,” have sub-sections (a nested structure) such as “Visual Assets”, “Audio Assets”, and “3D Models.”
Now, let’s see how we can use nested for loops in Liquid to dynamically generate the navigation menu for this documentation.
{% for mainItem in page.menu %}
<div>
<a href="#{{ mainItem.section.url }}">{{mainItem.section }}</a><br />
{% for item in mainItem.section.menu %}
<div>
<a href="#{{ item.section.url }}">{{ item.section }}</a><br />
</div>
{% endfor %}
</div>
{% endfor %}
Here’s a breakdown of what’s happening:
Outer Loop: The outer for loop iterates through each item in page.menu. page.menu refers to the menu data defined in our YAML front matter. mainItem represents each individual section (e.g., “Foundational Concepts”, “Development”). Main Section Link: Inside the outer loop, we create a link for the main section using {{ mainItem.section }} for the link text and {{ mainItem.url }} for the link’s href.
Conditional Check: The {% if mainItem.menu %} block checks if the current mainItem has a menu (i.e., sub-sections). This is crucial to avoid errors when a main section doesn’t have any nested items.
Inner Loop: If a menu exists, the inner for loop iterates through each item in mainItem.menu. item represents each sub-section (e.g., “Visual Assets”, “Audio Assets”).
Sub-section Link: Inside the inner loop, we create a link for the sub-section, similar to the main section link, using {{ item.section }} and {{ item.url }} .
Closing Tags: Make sure to close both the inner and outer for loops with {% endfor %} and the conditional check with {% endif %} .
This code snippet dynamically generates a nested navigation menu based on the structure defined in your YAML front matter. This makes it easy to manage and update your documentation structure without manually writing HTML for each link. The use of the conditional check ensures that the code gracefully handles sections without sub-sections, preventing errors and making your code more robust.
Targeting First and Last Items:
Sometimes, you need to apply special styling or logic to the first or last item in your loop. Liquid provides the forloop.first and forloop.last variables for this purpose. Here’s how you can use them:
{% for mainItem in page.menu %}
<div class="{% if forloop.first %}first-item{% endif %} {% if forloop.last %}last-item{% endif %}">
<a href="#{{ mainItem.url }}">{{ mainItem.section }}</a><br />
{% if mainItem.menu %}
{% for item in mainItem.menu %}
<div class="{% if forloop.first %}first-item{% endif %} {% if forloop.last %}last-item{% endif %}">
<a href="#{{ item.url }}">{{ item.section }}</a><br />
</div>
{% endfor %}
{% endif %}
</div>
{% endfor %}
In this enhanced code:
We’ve added class=”{% if forloop.first %} first-item {% endif %} {% if forloop.last %} last-item {% endif %}” to both the outer and inner loop’s div elements. This dynamically adds the class first-item to the first main section and the first sub-section within each main section. Similarly, it adds the class last-item to the last main section and the last sub-section within each main section. Now you can use CSS to style these elements differently. For example, you could add a different background color to the first and last menu items.
This technique is invaluable for various use cases, such as adding separators between list items (but not after the last item), applying special styling to the first or last element in a list, or conditionally rendering content for the first or last item.
By mastering nested loops and forloop.first/forloop.last in Liquid, you gain fine-grained control over your Jekyll templates, enabling you to create highly customized and dynamic websites. Remember to always close your if statements and for loops with {% endif %} and {% endfor %} respectively.
If you want me to continue writing interesting stuff you can support me via: